Why Do All My Purchases Continue to Fail in Roblox
A Developer Product is an item or ability that a user can purchase more than once, such as in-experience currency, ammo, or potions.
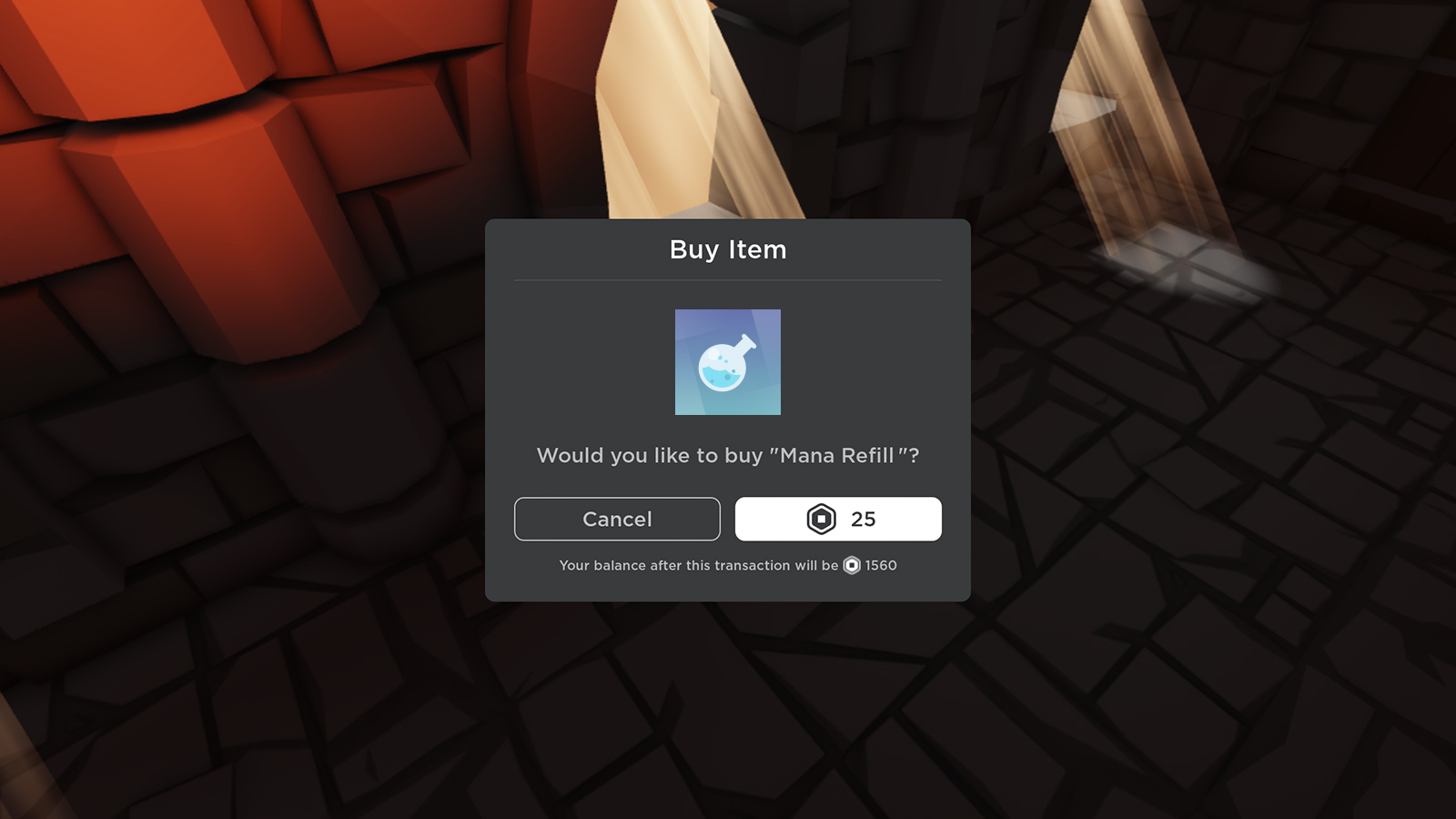
Creating Developer Products
You can create Developer Products directly in Studio.
To create a new Developer Product:
-
In the Home tab of the menu bar, click Game Settings. The Game Settings menu displays.
-
In the left-hand navigation of the Game Settings menu, click Monetization.
-
To the right of the Developer Products section, click the Create button. A placeholder Developer Product displays below Developer Products.
-
For the new placeholder product, click the … button and select Edit. The Edit Developer Product page displays.
-
Fill in the following fields:
-
Name: A title for your Developer Product.
-
Price: The amount of Robux you want to charge users for the Developer Product.
-
-
Click the Save button.
The new Developer Product is now available in the Developer Products section of the Monetization tab. You can reference this section to find all product IDs.

Scripting Developer Products
You must use scripting to implement Developer Product effects in your experiences.
Common Developer Product scripting use cases include:
Prompting Purchases
You can prompt a user to purchase one of your Developer Products with the MarketplaceService:PromptProductPurchase() method of MarketplaceService. Depending on the needs of your experience, you can call the MarketplaceService:PromptPurchase() function in situations such as when the user presses a button or when their character talks to a vendor NPC.
1 local MarketplaceService = game:GetService( "MarketplaceService" )
2 local Players = game:GetService( "Players" )
3 local player = Players.LocalPlayer
4
5 local productId = 0000000 -- Change this to your developer product ID
6
7 -- Function to prompt purchase of the developer product
8 local function promptPurchase ()
9 MarketplaceService:PromptProductPurchase(player, productId)
10 end
11
Handling Purchases
After a user purchases a Developer Product, it's your responsibility to handle and record the transaction. You can do this through a Script within ServerScriptService using the MarketplaceService.ProcessReceipt callback. The function you define will be called repetitively until it returns ProductPurchaseDecision.PurchaseGranted
1 local MarketplaceService = game:GetService( "MarketplaceService" )
2 local Players = game:GetService( "Players" )
3
4 local productFunctions = {}
5
6 -- ProductId 123123 brings the player back to full health
7 productFunctions[ 123123 ] = function (receipt, player)
8 if player.Character and player.Character:FindFirstChild( "Humanoid" ) then
9 player.Character.Humanoid.Health = player.Character.Humanoid.MaxHealth
10 -- Indicate a successful purchase
11 return true
12 end
13 end
14
15 -- ProductId 456456 awards 100 gold to the player
16 productFunctions[ 456456 ] = function (receipt, player)
17 local stats = player:FindFirstChild( "leaderstats" )
18 local gold = stats and stats:FindFirstChild( "Gold" )
19
20 if gold then
21 gold.Value = gold.Value + 100
22 return true
23 end
24 end
25
26 local function processReceipt (receiptInfo)
27 local userId = receiptInfo.PlayerId
28 local productId = receiptInfo.ProductId
29
30 local player = Players:GetPlayerByUserId(userId)
31 if player then
32 -- Get the handler function associated with the product ID and attempt to run it
33 local handler = productFunctions[productId]
34 local success, result = pcall (handler, receiptInfo, player)
35 if success then
36 -- The player has received their benefits!
37 -- return PurchaseGranted to confirm the transaction.
38 return Enum.ProductPurchaseDecision.PurchaseGranted
39 else
40 warn( "Failed to process receipt:" , receiptInfo, result)
41 end
42 end
43
44 -- the player's benefits couldn't be awarded.
45 -- return NotProcessedYet to try again next time the player joins.
46 return Enum.ProductPurchaseDecision.NotProcessedYet
47 end
48
49 -- Set the callback; this can only be done once by one script on the server!
50 MarketplaceService.ProcessReceipt = processReceipt
51
The receiptInfo table passed to the processReceipt() callback function contains detailed information on the purchase. For a list of keys and descriptions, see the ProcessReceipt API reference.
Getting Information
To get information about a specific Developer Product, such as its price, name, or image, use the MarketplaceService:GetProductInfo() function with a second argument of InfoType.Product. For example:
1 local MarketplaceService = game:GetService( "MarketplaceService" )
2
3 local productId = 000000 -- Change this to your developer product ID
4
5 local productInfo = MarketplaceService:GetProductInfo(productId, Enum.InfoType.Product)
6
7 local success, productInfo = pcall ( function ()
8 return MarketplaceService:GetProductInfo(productId, Enum.InfoType.Product)
9 end )
10
11 if success then
12 -- Use productInfo here!
13 end
14
You can also get the data for all of the developer products in an experience by using the MarketplaceService:GetDeveloperProductsAsync() method. This returns a Pages object that you can inspect and filter to build things like an in-experience store or product list GUI.
For example, the following script prints the name, price, ID, description, and AssetID for all Developer Products in an experience:
1 local MarketplaceService = game:GetService( "MarketplaceService" )
2
3 local success, developerProducts = pcall ( function ()
4 return MarketplaceService:GetDeveloperProductsAsync():GetCurrentPage()
5 end )
6
7 if developerProducts then
8 for _, developerProduct in pairs (developerProducts) do
9 for field, value in pairs (developerProduct) do
10 print (field .. ": " .. value)
11 end
12 print ( " " )
13 end
14 end
15
Source: https://create.roblox.com/docs/production/monetization/developer-products
0 Response to "Why Do All My Purchases Continue to Fail in Roblox"
Post a Comment